Node.js SDK
Last updated: Sep-12-2024
The Cloudinary Node.js SDK provides simple, yet comprehensive image and video upload, transformation, optimization, and delivery capabilities through the Cloudinary APIs, that you can implement using code that integrates seamlessly with your existing Node.js application.
How would you like to learn?
Resource | Description |
---|---|
Node.js quick start | Get up and running in five minutes with a walk through of installation, configuration, upload, management and transformations. |
Video tutorials | Watch tutorials relevant to your use cases, from getting started with the Node.js SDK, to uploading, transforming and analyzing your images and videos. |
Cloudinary Node.js SDK GitHub repo | Explore the source code and see the CHANGELOG for details on all new features and fixes from previous versions. |
![]() |
Try the free Introduction to Cloudinary for Node.js Developers online course, where you can learn how to upload, manage, transform and optimize your digital assets. |
Install
Cloudinary's Node.js integration library is available as an open-source NPM package. To install the library, run:
Configure
Include Cloudinary's Node.js classes in your code:
cloudinary.v2...
. In your own code, it is recommended to include v2
of the Node.js classes as follows:
Alternatively, from within a module, you can use an ES6 import statement:
Following either of these, your upload and Admin API calls should omit the .v2
shown in the code examples of this guide.
For example, a simple image upload:
Set required configuration parameters
You can set the required configuration parameters, cloud_name
, api_key
and api_secret
either using the CLOUDINARY_URL
environment variable, or using the config
method in your code.
To define the CLOUDINARY_URL
environment variable:
- Copy the API environment variable format from the API Keys page of the Cloudinary Console Settings.
- Replace
<your_api_key>
and<your_api_secret>
with your actual values. Your cloud name is already correctly included in the format.
For example:
Alternatively, you can use the config
method to set your cloud_name
, api_key
and api_secret
, for example:
- When writing your own applications, follow your organization's policy on storing secrets and don't expose your API secret.
- If you use a method that involves writing your environment variable to a file (e.g.
dotenv
), the file should be excluded from your version control system, so as not to expose it publicly.
Set additional configuration parameters
In addition to the required configuration parameters, you can define a number of optional configuration parameters if relevant.
You can append configuration parameters, for example upload_prefix
and secure_distribution
, to the environment variable:
Or you can use the config
method in your code, for example:
urlAnalytics
configuration option. Learn more.Configuration video tutorials
If you've had trouble with any of the above steps, you may find these video tutorials helpful:
Find your credentials
Find your Cloudinary credentials for APIs and SDKsConfigure the Node.js SDK
Install and configure the Cloudinary Node.js SDKSee more Node.js video tutorials.
Use
Once you've installed and configured the Node.js SDK, you can use it for:
- Uploading files to your product environment: You can upload any files, not only images and videos, set your own naming conventions and overwrite policies, moderate and tag your assets on upload, and much more.
- Transforming and optimizing images and videos: Keeping your original assets intact in your product environment, you can deliver different versions of your media - different sizes, formats, with effects and overlays, customized for your needs.
- Managing assets: Using methods from the Admin and Upload APIs, you can organize your assets, for example, list, rename and delete them, add tags and metadata and use advanced search capabilities.
Quick example: File upload
The following Node.js code uploads the dog.mp4
video using the public_id, my_dog
. The video will overwrite the existing my_dog
video if it exists. When the video upload is complete, the specified notification URL will receive details about the uploaded media asset.
- Read the Upload guide to learn more about customizing uploads, using upload presets and more.
- See more examples of image and video upload using the Cloudinary Node.js library.
- Explore the Upload API reference to see all available methods and options.
Quick example: Transform and optimize
Take a look at the following transformation code and the image it delivers:
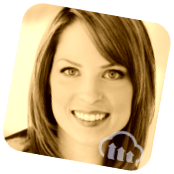
This relatively simple code performs all of the following on the original front_face.jpg image before delivering it:
- Convert and deliver the image in PNG format (the originally uploaded image was a JPG)
- Crop to a 150x150 thumbnail using face-detection gravity to automatically determine the location for the crop
- Round the corners with a 20 pixel radius
- Apply a sepia effect
- Overlay the Cloudinary logo on the southeast corner of the image (with a slight offset). The logo is scaled down to a 50 pixel width, with increased brightness and partial transparency (opacity = 60%)
- Rotate the resulting image (including the overlay) by 10 degrees
- Optimize the image to reduce the size of the image without impacting visual quality.
And here's the URL that would be included in the image tag that's automatically generated from the above code:
In a similar way, you can transform a video.
- Read the Transform and customize assets guide to learn about the different ways to transform your assets.
- See more examples of image and video transformations using the Cloudinary Node.js library.
- See all possible transformations in the Transformation URL API reference.
Quick example: Get details of a single asset
The following Node.js example uses the Admin API resource method to return details of the image with public ID cld-sample
:
Sample output:
- Check out the Manage and analyze assets guide for all the different capabilities.
- Get an overview of asset management using the Node.js SDK.
- Select the
Node.js
tab in the Admin API and Upload API references to see example code snippets.
Sample projects
For additional useful code samples and to learn how to integrate Cloudinary with your Node.js applications, take a look at our sample projects on GitHub.
- Basic Node.js sample: Uploading local and remote images to Cloudinary and generating various transformation URLs.
- Node.js Photo Album: A fully working web application that allows you to upload photos, maintain a database with references, list images with their metadata, and display them using various cloud-based transformations. Image uploading is performed both from the server side and directly from the browser using a jQuery plugin.
- Try out the Node.js SDK using the quick start.
- Learn more about uploading images and videos using the Node.js SDK.
- See examples of powerful image and video transformations using Node.js code, and see our image transformations and video transformation docs.
- Check out Cloudinary's asset management capabilities, for example, renaming and deleting assets, adding tags and metadata to assets, and searching for assets.
- Stay tuned for updates with the Programmable Media Release Notes and the Cloudinary Blog.